How to add a page to the WordPress admin sidebar menu
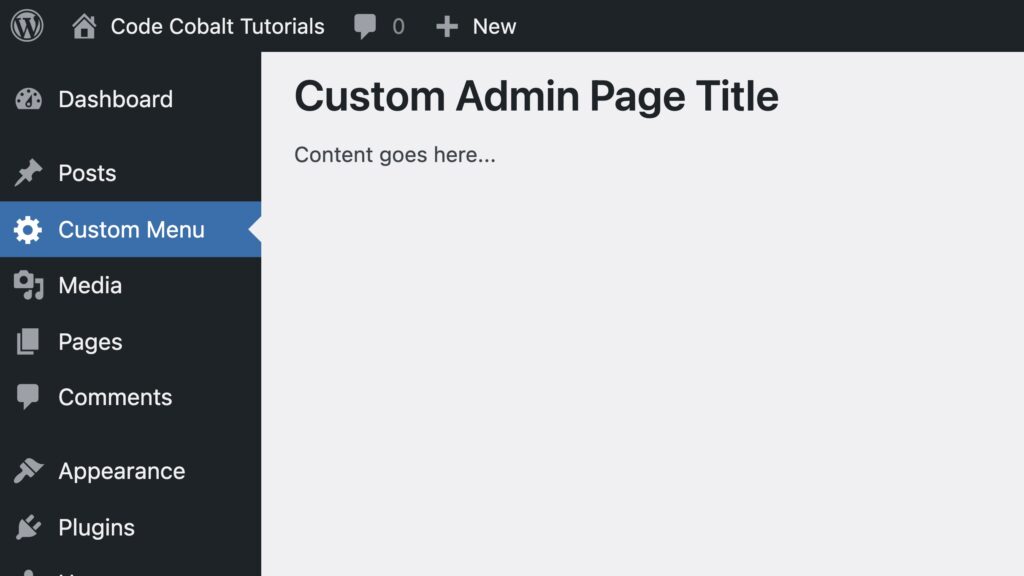
This is a brief guide on how to add a custom top-level menu item and corresponding admin screen to the WordPress admin sidebar.
The code in this tutorial can be added to your theme’s functions.php, added using a code snippets plugins like Code Snippets, or elsewhere in a custom plugin or child theme.
Step 1: Add admin menu hook
First, let’s add an action for the admin_menu
hook.
add_action('admin_menu', 'custom_admin_page');
function custom_admin_page()
{
add_menu_page(
'Custom Page Title',
'Custom Menu',
'manage_options',
'custom-admin-page',
'custom_admin_page_content',
'dashicons-admin-generic',
6
);
}
Code CobaltLet’s take a look at the options we have available in add_menu_page
. Here is the function prototype:
function add_menu_page(
$page_title,
$menu_title,
$capability,
$menu_slug,
$callback = '',
$icon_url = '',
$position = null
) {
Code Cobalt1. page_title – Title of the page used in the title tags.
2. menu_title – Text to be displayed in the sidebar.
3. capability – The capability
required for this menu item to be displayed. “manage_options”
is a good choice to restrict access to Administrators.
Read more at https://wordpress.org/documentation/article/roles-and-capabilities/
4. callback – The function that will output the content for the page.
5. icon_url – Optional icon for the class.
You can use icons here https://developer.wordpress.org/resource/dashicons/
6. position – A priority number to control the relative position of the element in the sidebar.
Read more here: https://developer.wordpress.org/reference/functions/add_menu_page/
Step 2: Output the contents of the page
Define the function in your callback from Step 1. Use any method to output the necessary HTML.
function custom_admin_page_content()
{
echo '<h1>Custom Admin Page Title</h1>';
echo '<p>Content goes here...</p>';
}
Code CobaltAnd we are done! You should now see your menu on the sidebar and your content should display when you select it.
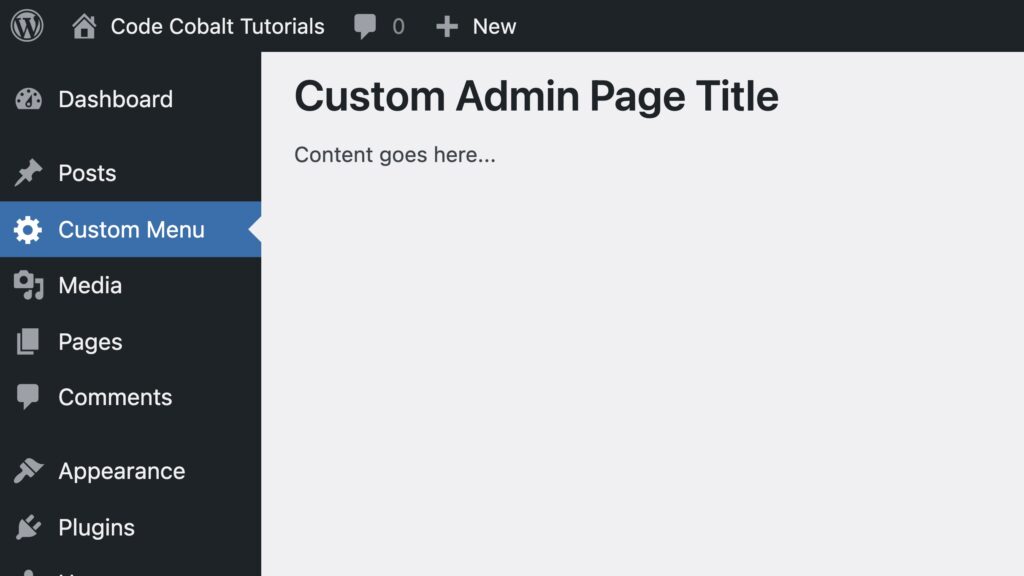
Full Example
add_action('admin_menu', 'custom_admin_page');
function custom_admin_page()
{
add_menu_page(
'Custom Page Title',
'Custom Menu',
'manage_options',
'custom-admin-page',
'custom_admin_page_content',
'dashicons-admin-generic',
6
);
}
function custom_admin_page_content()
{
echo '<h1>Custom Admin Page Title</h1>';
echo '<p>Content goes here...</p>';
}
Code Cobalt